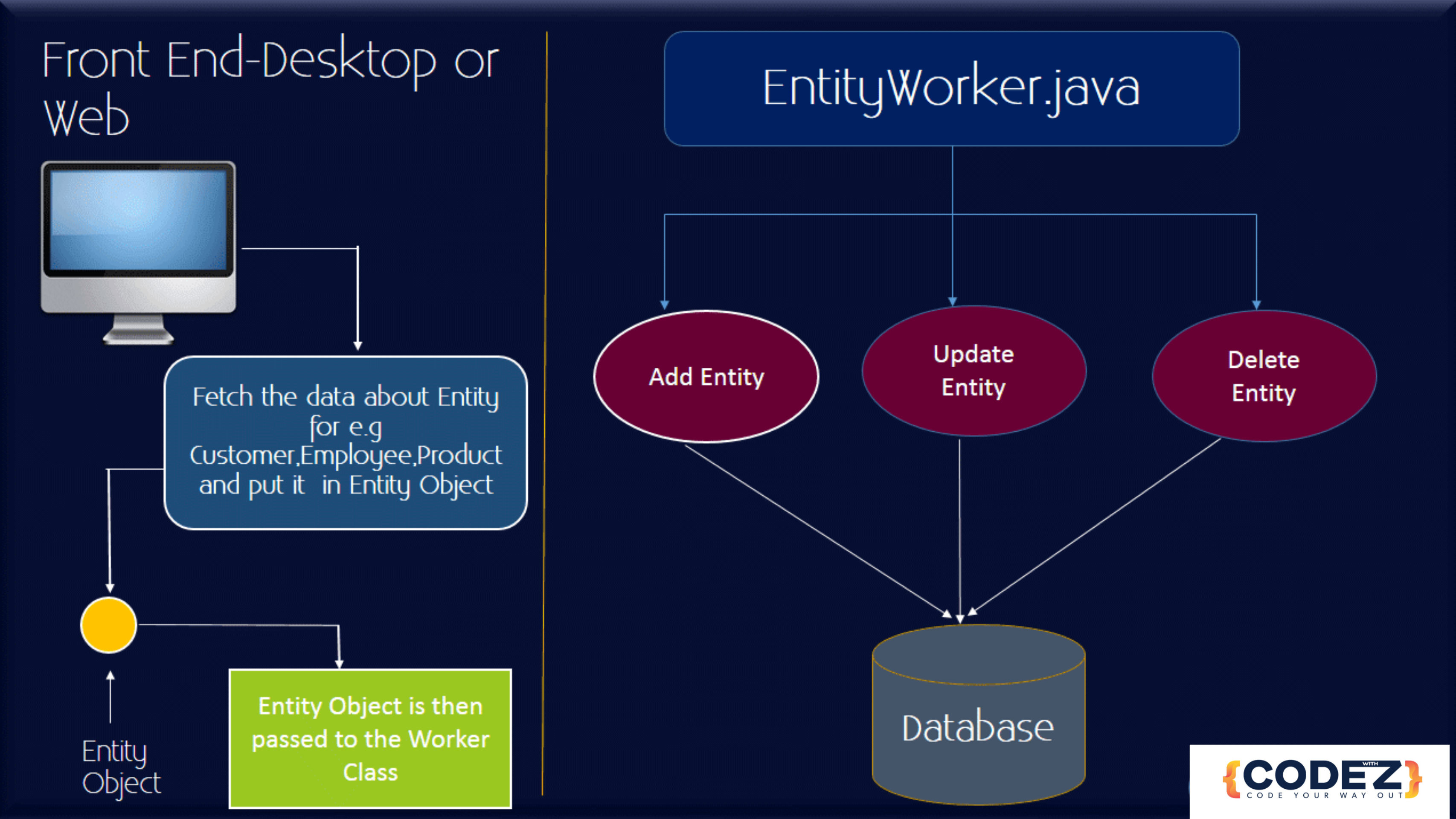
Structure of Worker Model |
Now as the image shows the flow. Let us create a Worker class for Customer.
So my table customer looks like this
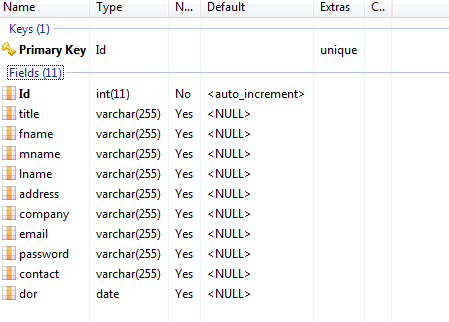
Structure of Customer Table |
- Now as our database table is ready we can develop our Entity class in this case it is Customer.java
- Entity Class basically contains the fields of the Entity and its setter and getter methods.
public class Customer
{
int id;
String fname,mname,lname,address,contact,email,password,dor,company,title;
public Customer() {
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFname() {
return fname;
}
public void setFname(String fname) {
this.fname = fname;
}
public String getMname() {
return mname;
}
public void setMname(String mname) {
this.mname = mname;
}
public String getLname() {
return lname;
}
public void setLname(String lname) {
this.lname = lname;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getContact() {
return contact;
}
public void setContact(String contact) {
this.contact = contact;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getDor() {
return dor;
}
public void setDor(String dor) {
this.dor = dor;
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
}
Now the last and important step in developing of this model is Worker file.
- Now i will name the worker as EntityWorker.java with three important methods
- addEntity(Entity e)
- updateEntity(Entity e)
- deleteEntity(int id)
So as per the model my worker will be named as CustomerWorker.java and methods would be
- addCustomer(Customer c)
- updateCustomer(Customer c)
- deleteCustomer(int id)
Now let us go in deep and understand a single method
Method will return String based on which we decide the flow.
public static String addCustomer(Customer c)
{
String result="";
try
{
String query="Insert into customer(title,fname,mname,lname,address,company,email,password,contact,dor) values (?,?,?,?,?,?,?,?,?,?);";
---> I have used the PreparedStatement here and to understand what is DatabaseConnector click on it.
Got all the data from customer object and set it in pstmt object and executed it.<---
PreparedStatement pstmt=DatabaseConnector.getPreparedStatement(query);
pstmt.setString(1,c.getTitle());
pstmt.setString(2,c.getFname());
pstmt.setString(3,c.getMname());
pstmt.setString(4,c.getLname());
pstmt.setString(5,c.getAddress());
pstmt.setString(6,c.getCompany());
pstmt.setString(7,c.getEmail());
pstmt.setString(8,c.getPassword());
pstmt.setString(9,c.getContact());
pstmt.setString(10,c.getDor());
int i=pstmt.executeUpdate();
if(i==1)
{
result="SUCCESS";
}
else
{
result="FAIL";
}
}
catch (Exception e)
{
result="PROBLEM";
e.printStackTrace();
}
return result;
}
So the overall CustomerWorker .java file will look like this.
CustomerWorker.java
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.Statement;
public class CustomerWorker
{
public static String addCustomer(Customer c)
{
String result="";
try
{
String query="Insert into customer(title,fname,mname,lname,address,company,email,password,contact,dor) values (?,?,?,?,?,?,?,?,?,?);";
PreparedStatement pstmt=DatabaseConnector.getPreparedStatement(query);
pstmt.setString(1,c.getTitle());
pstmt.setString(2,c.getFname());
pstmt.setString(3,c.getMname());
pstmt.setString(4,c.getLname());
pstmt.setString(5,c.getAddress());
pstmt.setString(6,c.getCompany());
pstmt.setString(7,c.getEmail());
pstmt.setString(8,c.getPassword());
pstmt.setString(9,c.getContact());
pstmt.setString(10,c.getDor());
int i=pstmt.executeUpdate();
if(i==1)
{
result=Results.SUCCESS;
}
else
{
result=Results.FAIL;
}
}
catch (Exception e)
{
result=Results.PROBLEM;
e.printStackTrace();
}
return result;
}
public static String updateCustomer(Customer c)
{
String result="";
try
{
String query="Update customer set title=?,fname=?,mname=?,lname=?,address=?,company=?,email=?,password=?,contact=?,dor=? where id=?;";
PreparedStatement pstmt=DatabaseConnector.getPreparedStatement(query);
pstmt.setString(1,c.getTitle());
pstmt.setString(2,c.getFname());
pstmt.setString(3,c.getMname());
pstmt.setString(4,c.getLname());
pstmt.setString(5,c.getAddress());
pstmt.setString(6,c.getCompany());
pstmt.setString(7,c.getEmail());
pstmt.setString(8,c.getPassword());
pstmt.setString(9,c.getContact());
pstmt.setString(10,c.getDor());
pstmt.setInt(11,c.getId());
int i=pstmt.executeUpdate();
if(i==1)
{
result=Results.SUCCESS;
}
else
{
result=Results.FAIL;
}
}
catch (Exception e)
{
result=Results.PROBLEM;
e.printStackTrace();
}
return result;
}
public static String deleteCustomer(int id)
{
String result="";
try
{
String query="Delete from customer where id=?;";
PreparedStatement pstmt=DatabaseConnector.getPreparedStatement(query);
pstmt.setInt(1,id);
int i=pstmt.executeUpdate();
if(i==1)
{
result=Results.SUCCESS;
}
else
{
result=Results.FAIL;
}
}
catch (Exception e)
{
result=Results.PROBLEM;
e.printStackTrace();
}
return result;
}
public static String checkLogin(String user,String password)
{
String result="";
String dbUser="",dbPass="";
try {
Statement st=DatabaseConnector.getStatement();
String query="Select email from customer where email like '"+user+"' and password like '"+password+"'; " ;
ResultSet rs=st.executeQuery(query);
if( rs.next())
{
result=Results.SUCCESS;
}
else
{
result=Results.FAIL;
}
}
catch (Exception e)
{
result=Results.PROBLEM;
e.printStackTrace();
}
return result;
}
}
CustomerWorker will have these 3 as well as additional methods for in later part.
These model can be used in both Desktop as well as Web Projects in Java.